Create a tariff in 5 mins
First, connect on Alkemics and go to your profile, create a new access keys.
Don't forget to copy your client secret before closing the modal, you won't be able to get it later
Let's first get the access token.
import json
import requests
# Data for authentication, update it with your own credential
authentication_payload = {
"client_id": "{your client id}", # replace with your client id
"client_secret": "{your client secret}", # replace with your client secret
"grant_type": "client_credentials",
}
# Call the authentication API
response = requests.post(
"https://apis.alkemics.com/auth/v2/token",
data=json.dumps(authentication_payload)
).json()
# Response contains:
# {"access_token": "{your access token}", "type": "JWT"}
access_token = response['access_token']
# We will use this access_token to access Alkemics APIS
headers = {
'Authorization': 'Bearer %s' % access_token,
'Content-Type': 'application/json', # We will be using json
}
Now that you have your access token, let's create a tariff and store its uuid
tariff = {
# data is an array because the API allows you to create/update
# multiple tariffs at once
"data": [
{
"data": {
"orderStartDate": "2019-05-17",
"targetCountryList": [
{"targetCountryCode": {"code": "250"}}
],
"priceWaterfallBasisQuantityNumber": [
{"data": 1, "expressedIn": {"code": "cnt"}}
],
"priceWaterfalls": [
{
"bracketUnit": {"code": "ctn"},
"brackets": [
{"min": 1},
{"min": 10},
],
"levels": [{
"type": {"code": "price"},
"items": [{
"description": "Gross price",
"type": {"code": "grossPrice"},
"unit": {"code": "$"},
"values": [
{"value": ""},
{"value": ""},
],
}],
}],
},
],
},
"name": "Tariff 2019",
"status": "DRAFT",
"targetOrganization": {
"gln": "{your recipient GLN}",
},
# "uuid": "..." # Set the uuid to update the tariff defined by the uuid
}
]
}
response = requests.post(
"https://apis.alkemics.com/public/v1/tariffs",
data=json.dumps(tariff),
headers=headers,
).json()
# The resonse contains the created tariffs in the same order as the request
# payload
tariff_uuid = response['data'][0]['uuid']
As represented on the platform:
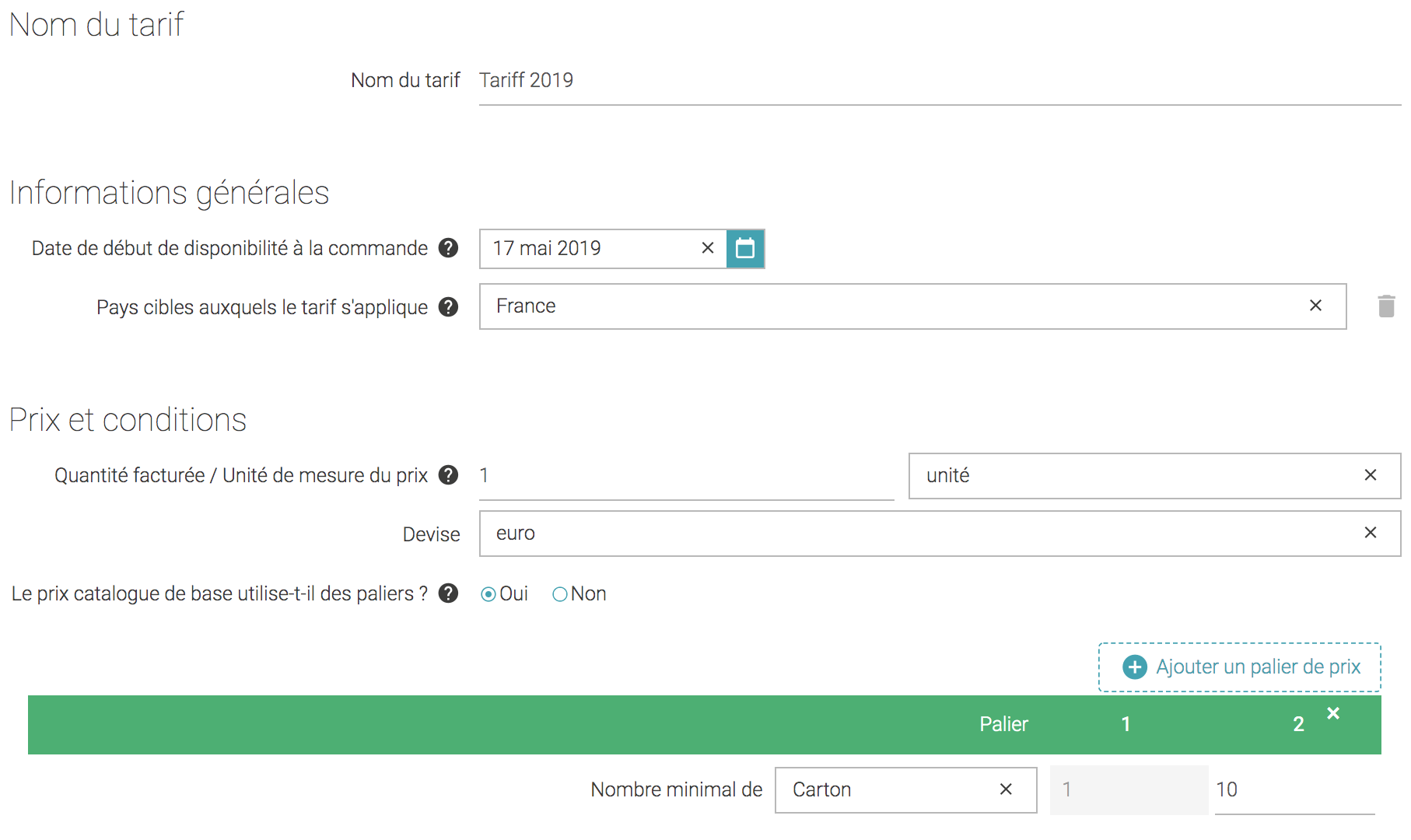
We will now link 2 products to this tariff:
- GTIN_1: this one is not yet in your catalog and hence will be created empty
- GTIN_2: this one is in your catalog. It has one logistical hierarchy with a pallet defined by GTIN_2_PALLET
products = {
'data': [{
'gtin': 'GTIN_1', # This product will be created
'suppliedId': '0123456', # Common between all the tariffs to which the product is linked
'data': { # Tariff-specific data for the product
'contractNumber': '1234-123456-12',
'priceWaterfalls': [{
'product': {'gtin': 'GTIN_1'},
'bracketUnit': {'code': 'ctn'},
'brackets': [
{'min': 1},
{'min': 10},
],
'levels': [{
'type': {'code': 'price'},
'items': [{
'description': 'Gross price',
'type': {'code': 'grossPrice'},
'unit': {'code': '$'},
'values': [
{'value': '10'},
{'value': '9'},
],
}],
}],
}],
},
}, {
'gtin': 'GTIN_2', # This product will be linked to the tariff
'suppliedId': '0123456',
'data': {
'contractNumber': '1234-123456-12',
'hierarchyProduct': {
'gtin': 'GTIN_2_PALLET',
},
'priceWaterfalls': [{
'product': {'gtin': 'GTIN_2'},
'bracketUnit': {'code': 'ctn'},
'brackets': [
{'min': 1},
{'min': 10},
],
'levels': [{
'type': {'code': 'price'},
'items': [{
'description': 'Gross price',
'type': {'code': 'grossPrice'},
'unit': {'code': '$'},
'values': [
{'value': '12'},
{'value': '10'},
],
}],
}],
}],
},
}]
}
requests.post(
"https://apis.alkemics.com/public/v1/tariffs/%s/products" % tariff_uuid,
data=json.dumps(products),
headers=headers,
).json()
The first product will be represented on the platform as:
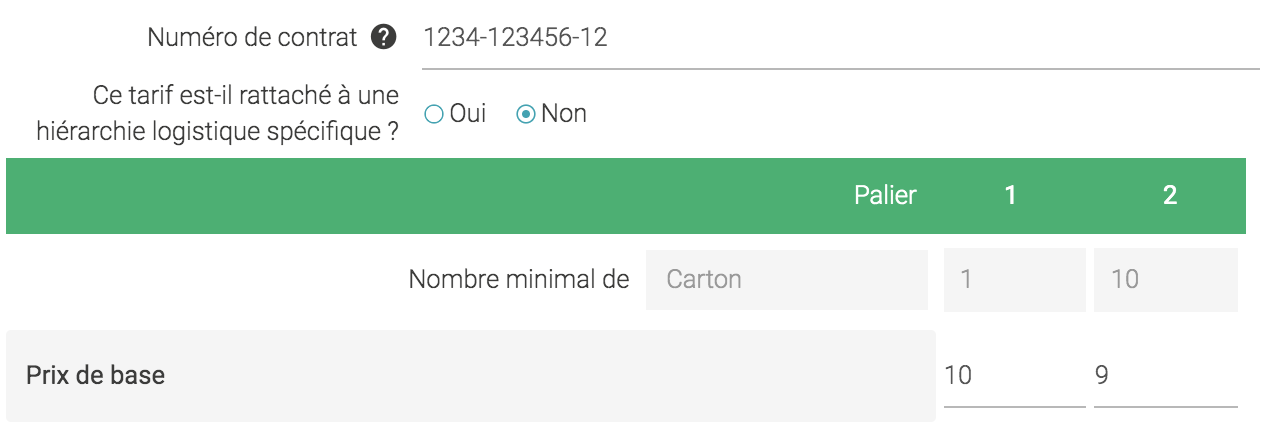
Let's say now that the target country filled when creating the tariff is not correct. We want to update it:
# Let's now update the target country:
tariff['data'][0]['uuid'] = tariff_uuid
tariff['data'][0]['data']['targetCountryList'][0]['targetCountryCode']['code'] = '250'
response = requests.post(
"https://apis.alkemics.com/public/v1/tariffs",
data=json.dumps(tariff),
headers=headers,
).json()
CongratulationsCheck out your tariffs on Alkemics, and you should see your newly created and updated tariff with 2 products linked to it.
Once everything is in order, and you no longer need to edit or add any products, you have to publish the tariff in order to make it available to the retailer
requests.post(
"https://apis.alkemics.com/public/v1/tariffs/%s/publish" % tariff_uuid,
data=json.dumps({}),
headers=headers,
).json()
WarningOnce a tariff is published, you can no longer edit it or add products to it.
If you want to add products, create a new one with those products
If you want to edit a field, you will need to archive the tariff and create a new one from scratch.
CongratulationsYou're tariff is now published to the retailer.
Updated about 1 month ago